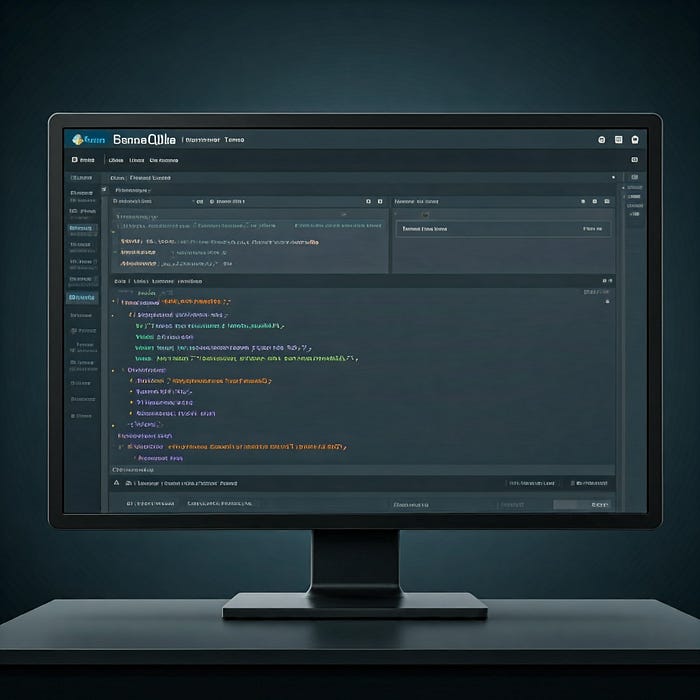
Setting Up Pipeline with Jenkins and SonarQube for a Spring Boot Project
In this article, we will walk through the setup of a Continuous Integration pipeline for a Spring Boot project using Jenkins and SonarQube. By the end of this tutorial, you’ll have a simple pipeline that compiles, tests, and analyzes a Spring Boot project using SonarQube and Jenkins, and builds a Docker image. For demonstration purposes, we’ll create a simple “Hello World” API.
Prerequisites:
- Basic knowledge of Spring Boot, Jenkins, and SonarQube.
- Java installed on your system.
- Docker installed and running on your machine.
Step 1: Create a Simple Spring Boot Project
First, create a Spring Boot project using Spring Initializr.
- Navigate to Spring Initializr.
- Choose the following options:
- Project: Maven
- Language: Java
- Spring Boot Version: 3.0+ (or latest)
- Dependencies: Spring Web
- Click “Generate” to download the project.
- Extract the project and open it in your favorite IDE (e.g., IntelliJ, VSCode).
In src/main/java/com/example/demo/DemoApplication.java
, update the code to create a simple "Hello World" REST API:
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
@RestController
public class HelloWorldController {
@GetMapping("/hello")
public String hello() {
return "Hello, World!";
}
}
}
Setup application.properties:
spring.application.name=spring-sonarqube
server.port: 5000
Run the project to ensure it’s working correctly:
mvn spring-boot:run
Access the API by navigating to http://localhost:5000/hello
. You should see the "Hello, World!" message.
Step 2: Install Jenkins And SonarQube
To set up Jenkins and SonarQube for a Spring Boot project with Docker, follow these steps:
1. Prerequisites
Ensure the following are installed on your machine:
- Docker (for containerization)
- Jenkins (for CI/CD)
- SonarQube (for code quality analysis)
- A Spring Boot project to integrate Jenkins and SonarQube with.
2. Pull Docker Images
Start by pulling the necessary Docker images for Jenkins and SonarQube:
# Pull Jenkins Docker image
docker pull jenkins/jenkins:lts
# Pull SonarQube Docker image
docker pull sonarqube:lts
3. Install and Setup Jenkins
3.1 Start Jenkins Container
Run Jenkins in a Docker container:
docker run -d --name jenkins -p 8080:8080 -p 50000:50000 -v jenkins_home:/var/jenkins_home jenkins/jenkins:lts
This command:
- Exposes Jenkins on port 8080.
- Mounts the Jenkins home directory for persistent data.
Once Jenkins is up, navigate to http://localhost:8080
. You’ll need the Jenkins initial admin password, which you can retrieve by running:
docker exec jenkins cat /var/jenkins_home/secrets/initialAdminPassword
3.2 Install Plugins
After logging into Jenkins:
- Install the required plugins:
- Pipeline
- Git
- SonarQube Scanner
3.3 Configure Jenkins
Go to Manage Jenkins → Global Tool Configuration, and set up:
- Java (point to your JDK installation path).
- Git (it should auto-detect).
- SonarQube Scanner (configure this after installing SonarQube).
4. Install and Setup SonarQube
4.1 Start SonarQube Container
Run SonarQube using Docker:
docker run -d --name sonarqube -p 9000:9000 sonarqube:lts
This will expose SonarQube on port 9000. Access SonarQube by navigating to http://localhost:9000
.
4.2 Configure SonarQube
- Log in using the default credentials (
admin/admin
). - Go to Administration → Security → Users and change the default password.
- Create a new project in SonarQube and generate a token for Jenkins integration.
5. Integrating Jenkins with SonarQube
5.1 Configure SonarQube in Jenkins
- In Jenkins, go to Manage Jenkins → Configure System.
- Scroll to SonarQube servers section and click on Add SonarQube.
- Provide:
- A name for the server.
- The SonarQube server URL (e.g.,
http://localhost:9000
). - Authentication token (from the token generated in SonarQube).
- Under Manage Jenkins → Global Tool Configuration, configure the SonarQube Scanner with its installation name (e.g.,
SonarQube Scanner
).
6. Configuring the Spring Boot Project
6.1 Add SonarQube Properties
In your Spring Boot project, configure sonar-project.properties
for SonarQube analysis:
Create a file sonar-project.properties
in the root directory of your project:
sonar.projectKey=spring-sonarqube
sonar.projectName=spring-sonarqube
sonar.projectVersion=1.0
sonar.sources=src/main/java
sonar.java.binaries=target
sonar.token=your_token
sonar.host.url=http://localhost:9000
6.2 Jenkins Pipeline Script
Add a Jenkinsfile
in the root of your Spring Boot project to define the CI/CD pipeline:
pipeline {
agent any
tools {
maven 'Maven 3.8.1' // Adjust the Maven version based on your setup
jdk 'JDK11' // Adjust the JDK version based on your setup
}
stages {
stage('Checkout') {
steps {
git 'https://github.com/your-repo/spring-boot-app.git'
}
}
stage('Build') {
steps {
sh 'mvn clean install'
}
}
stage('SonarQube Analysis') {
steps {
withSonarQubeEnv('SonarQube') {
sh 'mvn sonar:sonar'
}
}
}
stage('Quality Gate') {
steps {
timeout(time: 1, unit: 'HOURS') {
waitForQualityGate abortPipeline: true
}
}
}
}
post {
always {
junit 'target/surefire-reports/*.xml'
archiveArtifacts artifacts: 'target/*.jar', allowEmptyArchive: true
}
}
}
7. Running the Pipeline
- Push your Spring Boot project (with the
Jenkinsfile
) to a Git repository. - In Jenkins, create a new Pipeline project.
- Set the Pipeline definition to point to the Git repository.
- Run the pipeline to trigger the build, run the SonarQube analysis, and generate the report.
8. Verify the SonarQube Results
Once the pipeline runs successfully, navigate to SonarQube (e.g., http://localhost:9000
) and view the code quality analysis and report for your Spring Boot project.
By following these simple steps, you have successfully set up a Continuous Integrationpipeline using Jenkins and SonarQube for a Spring Boot project. This setup enables continuous integration, code quality analysis, and easy containerization with Docker.